Import additional product images as gallery images
In some cases merchants provide alternative image URLs for their products. These alternative image URLs will be stored in their own fields. This tutorial explains how to import those additional product images as gallery images for the product.
For example, take a look at this product:
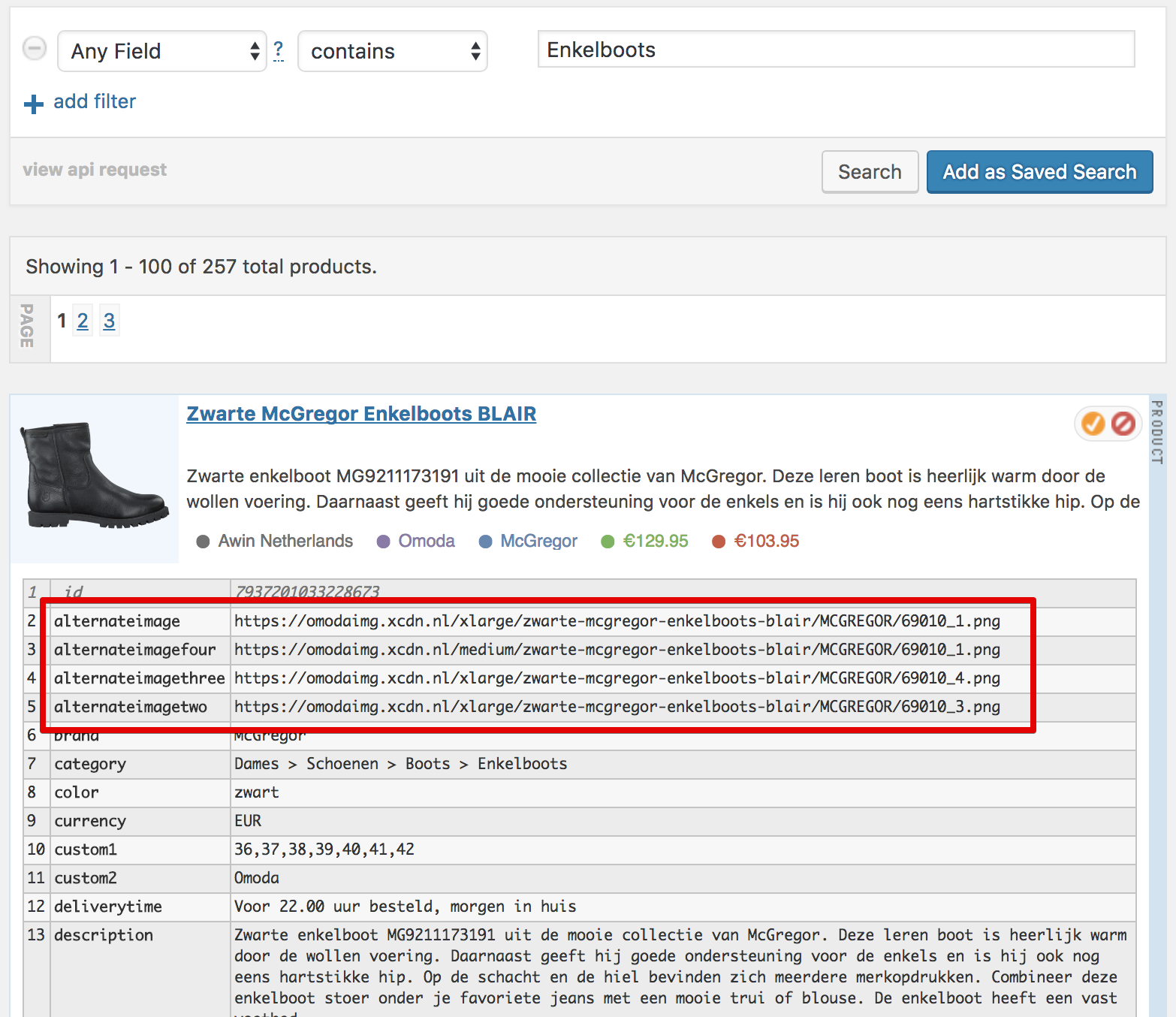
This product contains 4 additional product image URLs:
alternateimage
alternateimagefour
alternateimagethree
alternateimagetwo
Let's set up our code to import those 4 additional product images into our product image gallery so that our end result looks like this:
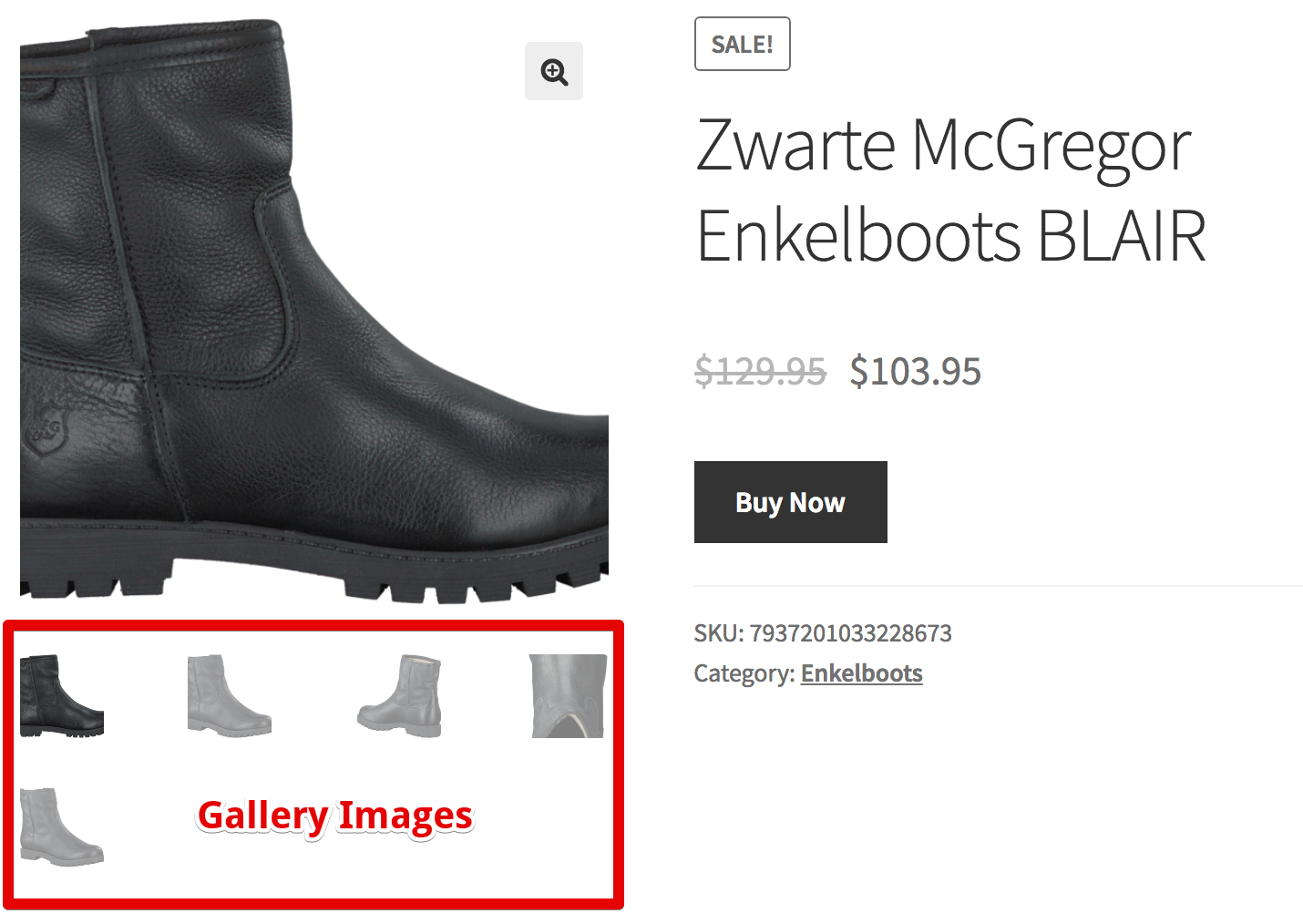
Heads-up!
Often times you might see alternate image URL fields for a product but those are just different sizes of the main image
field and NOT different images. Before adding the following code to your site, be sure that the additional image URLs are actually for different images, not just the same image at different sizes. Otherwise you will spend A LOT of time deleting duplicate images from your Media Library.
- 1
-
Create a custom plugin and add new feature flag
If you haven't done so already, create a custom plugin and add this code.
- 2
-
Add custom code
Add the following code to your custom plugin:
add_action( 'dfrpswc_post_save_product', function ( Dfrpswc_Product_Update_Handler $updater ) { /** * Modify this array and add the product fields that have alternate images * that you want imported into a product's gallery. */ $image_fields = [ 'alternateimage', 'alternateimagetwo', 'alternateimagethree', 'alternateimagefour', ]; /** STOP EDITING HERE **/ if ( ! function_exists( 'dfrapi_schedule_single_action' ) ) { return; } foreach ( $image_fields as $image_field ) { $post_id = $updater->wc_product->get_id(); dfrapi_schedule_single_action( date( 'U' ), 'mycode_import_product_gallery_image', compact( 'post_id', 'image_field' ), 'dfrpswc' ); } } ); add_action( 'dfrapi_as_mycode_import_product_gallery_image', function ( int $post_id, string $image_field ) { $wc_product = wc_get_product( $post_id ); if ( ! is_a( $wc_product, WC_Product::class ) ) { return; } $dfr_product = dfrps_product( $wc_product->get_id() ); if ( empty( $dfr_product ) ) { return; } if ( ! isset( $dfr_product[ $image_field ] ) ) { return; } if ( ! dfrapi_starts_with( $dfr_product[ $image_field ], [ 'http', '//' ] ) ) { return; } $gallery_meta_key = '_product_image_gallery'; $gallery_ids = get_post_meta( $wc_product->get_id(), $gallery_meta_key, true ); $gallery_ids = array_map( 'absint', array_filter( explode( ',', $gallery_ids ) ) ); $image_check_meta_key = '_mycode_product_image_gallery_' . $image_field; if ( dfrps_image_import_attempted( $wc_product->get_id(), $image_check_meta_key ) ) { return; } $image_data = dfrapi_image_data( $dfr_product[ $image_field ] ); $image_data->set_title( $wc_product->get_name() ); $image_data->set_filename( $wc_product->get_name() ); $image_data->set_description( $wc_product->get_name() ); $image_data->set_caption( $wc_product->get_name() ); $image_data->set_alternative_text( $wc_product->get_name() ); $image_data->set_author_id( dfrpswc_get_post_author_of_product_set_for_product( $wc_product->get_id() ) ); $image_data->set_post_parent_id( $wc_product->get_id() ); $image_data->set_post_thumbnail( false ); $uploader = dfrapi_image_uploader( $image_data ); $attachment_id = $uploader->upload(); update_post_meta( $wc_product->get_id(), $image_check_meta_key, 0 ); if ( ! is_wp_error( $attachment_id ) ) { $gallery_ids[] = $attachment_id; update_post_meta( $wc_product->get_id(), $gallery_meta_key, implode( ',', $gallery_ids ) ); update_post_meta( $attachment_id, '_owner_datafeedr', 'mycode' ); } }, 10, 2 );
- 3
-
Edit image fields
In the above code we have customized the image fields in these lines:
$image_fields = [ 'alternateimage', 'alternateimagetwo', 'alternateimagethree', 'alternateimagefour', ];
-
Your additional product image URL fields may have different field names. You should modify the
$image_fields
array to fit your needs. - 4
-
Save
Save your custom code.
Now the next time a product that contains those fields is updated during a Product Set update, their additional product images (if they exist) will be imported into the product gallery.